Please follow the links to the website to make the SQL Alchemy tutorial outline https://github.com/sqlalchemy/sqlalchemyChatGPT
Used MixerBox WebSearchG
SQLAlchemy tutorial outline
Based on the GitHub repository, the outline of a SQLAlchemy tutorial might look like this:
- Brief introduction
- What is SQLAlchemy?
- Key features and benefits
- Installation and setup
- How to install SQLAlchemy
- Initialize the settings
- Basic concepts
- ORM (Object Relational Mapping)
- Core SQL build system
- DBAPI interaction layer
- Create and manage database models
- Define the model
- Create and delete tables
- Query the system
- Base query
- Complex queries and subqueries
- Relationships and joins
- How to create a relationship
- How to perform the join operation
- Transaction management
- How to start and end transactions
- Error handling and rollback
- Performance optimization
- Eager loads
- Query optimization
- Database introspection and generation
- How to reflect the database schema
- Automatically generate CREATE statements
- Best practices and advanced topics
- security
- Performance tuning
This outline can serve as a basis for creating SQLAlchemy tutorials, especially for new IT learners and companies looking to develop their own management systems.
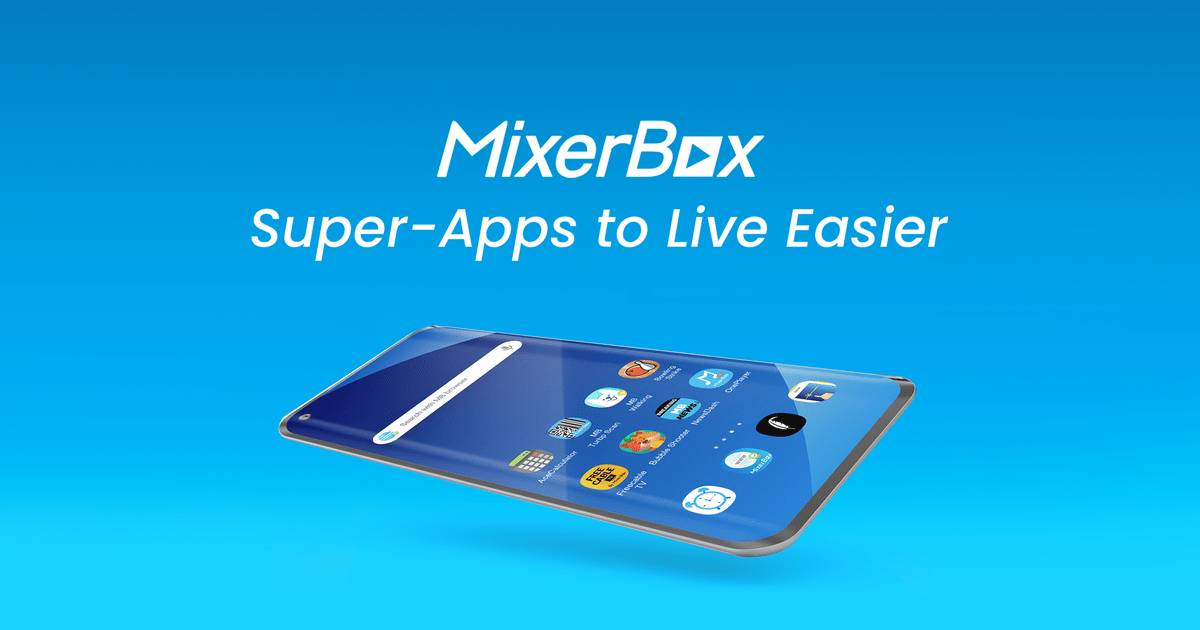
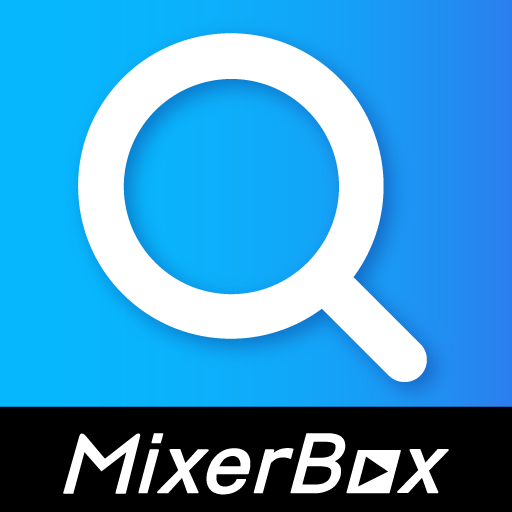
Please explain in detail what SQLAlchemy is? ChatGPT
What is SQLAlchemy?
SQLAlchemy is a database toolkit and Object-Relational Mapping (ORM) library for Python. It allows application developers to interact with databases in a Pythonic manner while providing the full power and flexibility of SQL. SQLAlchemy is designed to provide a set of enterprise-class persistence patterns for efficient and high-performance database access.
Main components
- ORM (Object Relational Mapping): SQLAlchemy’s ORM component allows developers to define database models using Python classes. These classes can be built and manipulated naturally, and all changes are automatically synchronized with the current transaction.
- Core SQL build system: This is another major component of SQLAlchemy, which is a complete database abstraction layer. It includes a Python-based SQL expression language, schema metadata, connection pooling, type enforcement, and custom types.
- DBAPI interaction layer: This layer handles low-level interaction with the database, including sending SQL queries and receiving results.
Key features
- Flexible query system: SQLAlchemy allows you to write queries using the same relational combination techniques that you use when writing SQL. While you can use literal SQL at any time, this is almost never needed.
- Eager loading: It provides a comprehensive and flexible eager loading system for loading related collections and objects.
- Transaction management: In the ORM of SQLAlchemy, no changes are persisted to storage until called.
commit()
- Database introspection and generation: The database schema can be reflected in a Python structure that represents the database metadata in one step; These structures can then generate CREATE statements.
- Security: It uses binding parameters as much as possible, making SQL injection attacks non-problematic.
Usage scenarios
- Web application backend
- Data analysis and data transformation tasks
- Automation scripts
- Enterprise applications
SQLAlchemy’s philosophy is that SQL databases and collections of objects behave less and less like when size and performance start to matter; Object collections behave less and less like tables and rows when abstraction begins to matter. SQLAlchemy is designed to accommodate these two principles, enabling developers to build a custom mediation layer between the domain model and the relational schema.
This library is ideal for developers and companies that need a flexible, efficient, and secure database solution.
Please explain in detail the main features and advantages of SQLAlchemy ChatGPT
SQLAlchemy Key Features and Benefits
Key features
- Object-relational mapping (ORM): SQLAlchemy’s ORM allows you to define and manipulate database models using Python classes. These classes correspond one-to-one to database tables, making database operations more intuitive and Pythonic.
- SQL Expression Language: SQLAlchemy Core provides a powerful SQL expression language that allows developers to build complex SQL queries in the Pythonic way.
- Database abstraction: Through DBAPI, SQLAlchemy provides a layer of database abstraction that allows code to easily switch between different database management systems (such as MySQL, PostgreSQL, SQLite, etc.).
- Eager loading: SQLAlchemy provides several eager loading options that improve query performance by allowing associated objects and collections to be preloaded in a query.
- Transaction management: SQLAlchemy supports complex transaction management, including nested transactions and savepoints, making error handling and data consistency more reliable.
- Data security: By using binding parameters and automatic escaping, SQLAlchemy helps prevent SQL injection attacks.
- Flexible query building: It provides a variety of ways to build queries, including chained methods and hybrid properties, to accommodate queries of varying complexity.
- Database migration and introspection: SQLAlchemy can “reflect” the database schema and generate the corresponding CREATE and DROP statements, which is useful for database migration.
advantage
- Flexibility: SQLAlchemy provides extreme flexibility, both at the ORM level and at the SQL expression language level, enabling virtually any type of database operation.
- Performance: SQLAlchemy performs well under high load through various optimizations, such as connection pooling and eager loading.
- Cross-database compatibility: Due to its database abstraction layer, applications that use SQLAlchemy can seamlessly switch between multiple databases without code changes.
- Community support and documentation: SQLAlchemy has a large community and rich documentation, which makes solving problems and learning relatively easy.
- Maturity and stability: SQLAlchemy has been around for many years and has been widely used in several large-scale projects, proving its maturity and stability.
- Full control: Unlike some more automated ORMs, SQLAlchemy gives you full control over the generation and execution of SQL queries, which is useful in situations where you need to manually optimize query performance.
- Advanced features: Advanced ORM features such as polymorphic associations, data map inheritance, and mixed attributes are supported.
- Testing and debugging: Provides tools and options for query analysis, performance testing, and debugging.
With these features and benefits, SQLAlchemy is suitable not only for simple CRUD applications, but also for complex enterprise database solutions. This makes it the library of choice for Python developers when it comes to database operations.
Please explain in detail how to install SQLAlchemyChatGPT
How to install and set up SQLAlchemy
Installing and setting up SQLAlchemy is a relatively simple and straightforward process. Here are the detailed steps:
Installation
- The easiest way to install using pip is to use Python’s package manager pip. Open a terminal or command prompt and run the following command: bashCopy code, which will download and install the latest version of SQLAlchemy.
pip install SQLAlchemy
- If you use Anaconda to manage your Python environment, you can also use conda to install SQLAlchemy:bashCopy code
conda install sqlalchemy
- If you need the latest development version or want to modify the source code of SQLAlchemy, you can clone and install from the GitHub repository: bashCopy code
git clone https://github.com/sqlalchemy/sqlalchemy.git cd sqlalchemy python setup.py install
Set up
- Import SQLAlchemy In your Python script or interactive environment, import the SQLAlchemy module to start using it. pythonCopy code
import sqlalchemy
- Create a database engineSQLAlchemy uses an Engine object to interact with the database. You can create an Engine like this: pythonCopy code, here, is the database dialect (e.g., , , etc.), and is the database credentials, and is the address and port of the database server, which is the database name to use.
from sqlalchemy import create_engine engine = create_engine('dialect://username:password@host:port/database')
dialect
mysql
postgresql
sqlite
username
password
host
port
database
- Creating Models and Tables Using SQLAlchemy’s ORM feature, you can define Python classes as database models and use those models to create database tables. pythonCopy code creates a table: pythonCopy code
from sqlalchemy import Column, Integer, String from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String)
Base.metadata.create_all(engine)
- Session management uses SQLAlchemy’s classes to manage database sessions. pythonCopy code
Session
from sqlalchemy.orm import Session session = Session(bind=engine)
With the above installation and setup steps, you should be able to start using SQLAlchemy for database operations. These are the most basic steps, and SQLAlchemy has more advanced features and setup options that can be configured to suit your specific needs.
Please explain in detail the initial settings for installing and setting up ChatGPT
SQLAlchemy initialization settings
Initialization setup is a series of configuration steps that occur after SQLAlchemy is installed to properly interact with the database. These settings typically involve creating the database engine, defining the data model, and initializing the session. Here are the detailed initialization setup steps:
Create a database engine
- To import the necessary modules, first, you need to import the function. pythonCopy code
create_engine
from sqlalchemy import create_engine
- Create Engine Object Use the function to create an Engine object. This object will be used for all interactions with the database. pythonCopy code
create_engine
engine = create_engine('dialect://username:password@host:port/database')
dialect
: Database dialects, such as , , , etc.mysql
postgresql
sqlite
username
and : Database login credentials.password
host
and : Database server address and port.port
database
: The name of the database to connect to.
定义数据模型
- 导入模块导入用于定义列和数据类型的模块。pythonCopy code
from sqlalchemy import Column, Integer, String
- 定义基类使用 函数创建一个基类。pythonCopy code
declarative_base
from sqlalchemy.ext.declarative import declarative_base Base = declarative_base()
- Create a data model that defines one or more Python classes that inherit from the base class. These classes are mapped to database tables. pythonCopy code
class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String)
Initialize the database session
- Import the Session class pythonCopy code
from sqlalchemy.orm import Session
- Creating a Session Object Use the Class to create a new Session object with the Engine object you created earlier as a parameter. pythonCopy code
Session
session = Session(bind=engine)
This completes the basic initialization setup of SQLAlchemy. You can now use this Session object for CRUD (create, read, update, delete) operations, or use the Engine object to execute raw SQL queries.
These are the most basic initialization settings. Depending on your needs, you can also make more advanced configurations, such as connection pooling settings, transaction isolation levels, and so on. However, these basic settings are usually sufficient for most common database operations.
Please explain in detail the basic concepts of ORM (Object Relational Mapping) ChatGPT
ORM (Object Relational Mapping): Basic concepts
What is ORM?
Object-Relational Mapping (ORM) is a programming technique used to create a viable virtual object database between an object-oriented programming language and a relational database. In SQLAlchemy for Python, ORM allows you to use Python classes called models to represent and manipulate database tables.
Main components
- Model: In ORM, a model is a Python class that defines how to create a table in a database. Each model corresponds to a table in the database.
- Session: A session is a unit of work that handles persistent operations. You can add, modify, and delete instances of a model through a session.
- Query: The query object is used to retrieve instances of the model from the database. In SQLAlchemy, you can use rich APIs to build complex SQL queries.
How does it work?
- Declaring a model: First, you need to define a model. This is usually a Python class inherited from . pythonCopy code
Base
from sqlalchemy import Column, Integer, String from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String)
- Create Tables: Once the model is defined, you can use the method to create database tables. pythonCopy code
create_all
Base.metadata.create_all(engine)
- Create Session: Create a session to start interacting with the database. pythonCopy code
from sqlalchemy.orm import Session session = Session(bind=engine)
- Persisted operations: CRUD operations using sessions.
- Add record pythonCopy code
new_user = User(name='John') session.add(new_user) session.commit()
- Query record pythonCopy code
users = session.query(User).filter_by(name='John').all()
- Update record pythonCopy code
user = session.query(User).filter_by(name='John').first() user.name = 'Johnny' session.commit()
- Delete the record pythonCopy code
user = session.query(User).filter_by(name='Johnny').first() session.delete(user) session.commit()
- Add record pythonCopy code
merit
- Abstraction layer: ORM provides a high-level abstraction layer that allows you to manipulate the database in an object-oriented manner rather than SQL statements.
- Database agnostic: Due to the abstraction of ORM, applications can easily switch between different database management systems.
- Security: ORM frameworks typically handle security issues such as SQL injection.
- Maintainability and readability: Using an ORM can make your code easier to maintain and read.
- DRY (Don’t Repeat Yourself): ORM allows you to define a data model in one place and then reuse it in multiple places, in line with DRY principles.
- Transaction management: ORMs often provide powerful transaction management capabilities that make complex operations more reliable.
By understanding these foundational concepts of ORM, you will be able to more effectively use SQLAlchemy or other ORM frameworks for database operations.
Please explain in detail the underlying concepts of the Core SQL build system ChatGPT
Core SQL Build System: Basic Concepts
What is the Core SQL build system?
In SQLAlchemy, the Core SQL build system is an underlying database abstraction layer that provides a set of Python APIs to build and execute SQL statements. Unlike the ORM layer, the Core SQL build system is closer to the original SQL while providing more flexibility and control.
Main components
- Engine: Engine is a core component of SQLAlchemy Core and is responsible for maintaining connections to databases.
- Dialect: Dialect is a subcomponent of Engine that handles SQL dialects and DBAPIs for specific database systems such as MySQL, PostgreSQL, etc.
- Expression Language: This is a Python DSL (Domain Specific Language) for building SQL queries.
- MetaData: The MetaData object is used to store all information related to the database schema (such as tables, columns, constraints, and so on).
- Table: In SQLAlchemy Core, the Table object is used to represent a table in a database.
- Column and Data Types: The Column object represents a field in a table, and the Data Types represents the data type of the field.
How does it work?
- Create EnginepythonCopy code
from sqlalchemy import create_engine engine = create_engine('dialect://username:password@host:port/database')
- Define MetaData and TablepythonCopy code
from sqlalchemy import MetaData, Table, Column, Integer, String metadata = MetaData() users = Table('users', metadata, Column('id', Integer, primary_key=True), Column('name', String) )
- Build and execute SQL queries
- Select (SELECT) pythonCopy code
from sqlalchemy import select s = select([users]) result = engine.execute(s)
- INSERT pythonCopy code
from sqlalchemy import insert i = insert(users).values(name='John') engine.execute(i)
- UPDATE pythonCopy code
from sqlalchemy import update u = update(users).where(users.c.name == 'John').values(name='Johnny') engine.execute(u)
- Delete pythonCopy code
from sqlalchemy import delete d = delete(users).where(users.c.name == 'Johnny') engine.execute(d)
- Select (SELECT) pythonCopy code
merit
- Flexibility: The Core SQL build system provides a very flexible way to build almost any type of SQL query.
- Control: It allows you to have more control, especially when you need to manually optimize queries or execute complex SQL logic.
- Database agnostic: Just like ORM, the Core SQL build system is database agnostic.
- Combinability: You can easily combine different SQL expressions and operations to create complex queries.
- Reusability: Since queries are built in the form of Python objects, you can easily reuse or extend them.
- Security: Similar to ORM, the Core SQL build system also provides a security mechanism to prevent SQL injection.
By understanding these foundational concepts of the SQLAlchemy Core SQL build system, you’ll be able to build and optimize database queries more effectively, especially if you need more flexibility and control.
Please explain in detail the basic concepts of DBAPI interaction layer ChatGPT
DBAPI Interaction Layer: Foundational Concepts
What is the DBAPI interaction layer?
The DBAPI (Database API) interaction layer is an underlying component in SQLAlchemy that is responsible for communicating directly with the database. This layer handles the execution of SQL queries and the return of result sets, as well as the beginning and end of transactions. It is an implementation of the Python DBAPI 2.0 specification for interacting with various databases.
Main components
- Connection Pool: Connection pools manage connections to databases to improve performance and resource utilization.
- The Cursor: Cursor object is used to execute SQL queries and get results.
- Transaction: This part is responsible for the start, commit, and rollback of transactions.
- Error Handling: The DBAPI layer is also responsible for error handling, including SQL errors and database connectivity issues.
How does it work?
- To get a connection in the DBAPI interaction layer, the first step is usually to get a database connection from the connection pool. pythonCopy code
connection = engine.connect()
- Execute SQL query Executes SQL query using the obtained connection. pythonCopy code or use parameterized queries for added security: pythonCopy code
result = connection.execute("SELECT * FROM users WHERE name = 'John'")
result = connection.execute("SELECT * FROM users WHERE name = :name", {'name': 'John'})
- Processing resultsYou can iterate through the result set to get all the rows returned by the query. pythonCopy code
for row in result: print(row)
- Transaction management is at the DBAPI layer, and you can also manage transactions manually. pythonCopy code
trans = connection.begin() try: connection.execute("INSERT INTO users (name) VALUES ('John')") trans.commit() except: trans.rollback()
- Close the connection when you are finished, close the database connection. pythonCopy code
connection.close()
merit
- Performance: Because DBAPI is a low-level interface, it generally provides better performance.
- Flexibility: It allows you to execute any SQL query, no matter how complex.
- Control: The DBAPI layer provides more control than other parts of SQLAlchemy, especially in transaction management and error handling.
- Direct access: If you need direct access to the database to perform special operations or optimize queries, DBAPI is a good choice.
- Cross-database: Because of the DBAPI 2.0 specification, this tier is also database-independent.
Notes
- Security: When using the DBAPI layer, you need to pay more attention to preventing SQL injection attacks.
- Complexity: This layer is often more difficult to use and maintain than ORM and Core SQL build systems.
By understanding these foundational concepts of SQLAlchemy’s DBAPI interaction layer, you will be able to perform low-level database operations more efficiently, especially if you need to interact directly with the database or manage complex transactions.
Please explain in detail the definition model for creating and managing database models ChatGPT
Create and manage database models: Define models
What is a database model?
In SQLAlchemy’s ORM (Object Relational Mapping), the database model is typically a Python class that defines the structure and behavior corresponding to the database tables. This class typically inherits from SQLAlchemy’s class and uses class properties to define table columns and other constraints.Base
Main components
- Base Class: All models should inherit from a base class, usually created through the method. pythonCopy code
declarative_base()
from sqlalchemy.ext.declarative import declarative_base Base = declarative_base()
- Table Name: Each model class should have a property that specifies the corresponding database table name.
__tablename__
- Columns: Use the Columns class to define the columns of a table and specify data types and other constraints.
Column
- Relationships: Use functions to define relationships between models, such as one-to-many, many-to-many, and so on.
relationship
How is a model defined?
- Import the necessary module pythonCopy code
from sqlalchemy import Column, Integer, String, ForeignKey from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import relationship
- Create the base class pythonCopy code
Base = declarative_base()
- Define the model class pythonCopy code
class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) email = Column(String, unique=True) # One-to-many relationship orders = relationship("Order", back_populates="user")
- Define association modelsIf you have models that have relationships with other tables, you can also define them in the same or different Python classes. pythonCopy code
class Order(Base): __tablename__ = 'orders' id = Column(Integer, primary_key=True) total = Column(Integer) user_id = Column(Integer, ForeignKey('users.id')) # Back-populate user = relationship("User", back_populates="orders")
Constraints and other options
- Primary Key: Marks the primary key with .
primary_key=True
- Unique: Use to ensure the uniqueness of the column.
unique=True
- Index: Use to create an index on the column to speed up queries.
index=True
- Default Values: Use parameters to set default values for columns.
default
- Nullable: Use to ensure that the column cannot contain NULL values.
nullable=False
Create a database table
Once all models are defined, you can use the method to create database tables.create_all
pythonCopy code
Base.metadata.create_all(engine)
This creates database tables based on all models defined.
merit
- Abstraction: By defining the model, you can interact with the database at a higher level of abstraction.
- Serviceability: Use models to make code easier to understand and maintain.
- Data integrity: By defining constraints and relationships at the model level, you can ensure the integrity of your data.
By learning how to define models in SQLAlchemy, you will be able to create and manage database models more efficiently, simplifying database operations and improving code quality.
Please explain in detail the creation and deletion tables for creating and managing database models ChatGPT
Create and manage database models: Create and drop tables
Create a database table
In SQLAlchemy, once you have defined database models (i.e. Python classes), the next step is usually to create database tables based on those models. This process typically involves the following steps:
- Import the necessary modules and model pythonCopy code
from sqlalchemy import create_engine from your_model_file import YourModel, Base # 假设你的模型在 'your_model_file' 文件中
- Create the database engine pythonCopy code
engine = create_engine('dialect://username:password@host:port/database')
- Using the
create_all
Method method creates a database table from all defined models. pythonCopy code This command checks whether a table corresponding to the model already exists in the database, and if not, creates a new table.create_all
Base.metadata.create_all(engine)
Delete the database table
Dropping a database table is a cautious step because it permanently deletes the table and all of its data. In SQLAlchemy, you can use the or method to drop a table.drop_all
drop
- Using the
drop_all
method deletes all database tables associated with . pythonCopy code warning: This will drop all tables, not just one.drop_all
Base
Base.metadata.drop_all(engine)
- Use the
drop
method If you want to drop only one specific table, you can use the Object method. pythonCopy code Here, it’s the model class for the table you want to delete.Table
drop
YourModel.__table__.drop(engine)
YourModel
Notes
- Backup: Before deleting any tables, make sure you have backed up all important data.
- Dependencies: Before dropping a table, make sure that no other tables depend on it, or that you are prepared to drop those dependent tables as well.
- Transactions: Consider performing a delete operation in a transaction so that you can roll back to a previous state if something goes wrong.
- Permissions: Ensure that you have sufficient permissions to create or delete tables.
By learning how to create and drop tables in SQLAlchemy, you will be able to manage your database model more effectively. These operations are often foundational steps in the database application development process, but they are also very important and sensitive and should be performed with caution.
Please explain in detail the basic query ChatGPT of the query system
Query system: Basic query
What is a base query?
In SQLAlchemy’s ORM, the underlying queries are primarily made through objects and classes. These queries are typically used to retrieve, filter, and sort data from a database.Session
Query
Main components
- The Session: object is the starting point for all ORM operations, including queries. pythonCopy code
Session
from sqlalchemy.orm import Session session = Session(bind=engine)
- Query Object: The Query Object: object is used to build and execute database queries. pythonCopy code
Query
query = session.query(YourModel)
The underlying query operation
- Select all records pythonCopy code
all_records = session.query(YourModel).all()
- Select a record pythonCopy code or pythonCopy code
first_record = session.query(YourModel).first()
one_record = session.query(YourModel).filter_by(id=1).one()
- Filter records using the or method. pythonCopy code or pythonCopy code
filter
filter_by
filtered_records = session.query(YourModel).filter(YourModel.name == 'John').all()
filtered_records = session.query(YourModel).filter_by(name='John').all()
- Sort records using the method. pythonCopy code
order_by
sorted_records = session.query(YourModel).order_by(YourModel.name).all()
- Count using the method. pythonCopy code
count
record_count = session.query(YourModel).count()
- Pagination uses the and methods. pythonCopy code
limit
offset
paginated_records = session.query(YourModel).limit(10).offset(20).all()
- Aggregate functions such as , , etc. pythonCopy code
sum
avg
from sqlalchemy import func total = session.query(func.sum(YourModel.field)).scalar()
Chained calls
You can chain multiple query methods together to build more complex queries.
pythonCopy code
complex_query = session.query(YourModel).filter_by(name='John').order_by(YourModel.id).limit(10).all()
merit
- Abstraction: With SQLAlchemy’s query system, you can perform complex database operations without writing SQL code directly.
- Readability and maintainability: Using Pythonic’s APIs and chained calls makes code easier to read and maintain.
- Security: SQLAlchemy’s query system helps prevent SQL injection attacks.
By understanding the underlying query operations of SQLAlchemy, you will be able to retrieve information from the database more efficiently without having to write SQL code directly. This not only improves the readability and maintainability of the code, but also increases the security of the application.
Please explain in detail the complex queries and subqueries of the query system ChatGPT
Query system: Complex queries and subqueries
Complex queries
In SQLAlchemy, in addition to the basic query, you can also execute more complex queries, including joins, group by, subqueries, and so on.
- Joins uses the Table join method. pythonCopy code or use to make outer joins. pythonCopy code
join
from sqlalchemy.orm import joinedload query = session.query(User).join(Order).filter(Order.amount > 100)
outerjoin
query = session.query(User).outerjoin(Order).filter(Order.amount == None)
- Group By uses the method to group it. pythonCopy code
group_by
from sqlalchemy import func query = session.query(User.name, func.count(Order.id)).join(Order).group_by(User.name)
- The Having clause uses the HAVING method to add a HAVING clause. pythonCopy code
having
query = session.query(User.name, func.count(Order.id)).join(Order).group_by(User.name).having(func.count(Order.id) > 2)
Subqueries
Subqueries are queries nested within other queries. In SQLAlchemy, you can use the method to create subqueries.subquery
- Scalar quantum query A scalar quantum query returns a single value. For example, find out the last order for each user. pythonCopy code
from sqlalchemy import desc subq = session.query(func.max(Order.date)).filter(Order.user_id == User.id).scalar_subquery() query = session.query(User, subq.label("last_order_date"))
- Association subqueriesAssociation subqueries are used in the main query, typically with operators such as , , or . pythonCopy code
exists
any
all
from sqlalchemy import exists subq = session.query(Order.id).filter(Order.user_id == User.id, Order.amount > 100).exists() query = session.query(User).filter(subq)
- Subquery as columns: You can also add a subquery as one or more columns to the main query. pythonCopy code
subq = session.query(func.count(Order.id)).filter(Order.user_id == User.id).label("total_orders") query = session.query(User, subq).group_by(User.id)
Chained calls
As with the underlying query, you can also chain multiple complex query methods together.
pythonCopy code
query = session.query(User).join(Order).filter(Order.amount > 100).group_by(User.name).having(func.count(Order.id) > 2)
merit
- Flexibility: Complex queries and subqueries provide extreme flexibility, allowing you to perform almost any type of database query.
- Optimization: Using subqueries and joins, you can optimize data retrieval and reduce network transfers and database load.
- Composable: You can combine basic and complex queries to meet specific data retrieval needs.
By understanding SQLAlchemy’s complex queries and subqueries, you’ll be able to build more advanced and flexible database queries to retrieve and analyze data more efficiently. These advanced features make SQLAlchemy a very powerful tool for database interaction.
Please explain in detail how relationships and joins create relationships in ChatGPT
Relationships and joins: How to create relationships
What is a relationship?
In a database, a relationship is a logical connection between two or more tables. In the ORM of SQLAlchemy, you can use functions to define relationships between models, such as one-to-one, one-to-many, and many-to-many.relationship
Main components
- ForeignKey: In SQLAlchemy, use classes to define foreign keys. pythonCopy code
ForeignKey
from sqlalchemy import ForeignKey user_id = Column(Integer, ForeignKey('users.id'))
- relationship: Use functions to define logical relationships between models. pythonCopy code
relationship
from sqlalchemy.orm import relationship orders = relationship("Order", back_populates="user")
How do I create a relationship?
- A one-to-one relationshipIn a one-to-one relationship, each row in two tables has and only one association with a row in the other table. pythonCopy code
class Parent(Base): __tablename__ = 'parents' id = Column(Integer, primary_key=True) child = relationship("Child", uselist=False, back_populates="parent") class Child(Base): __tablename__ = 'children' id = Column(Integer, primary_key=True) parent_id = Column(Integer, ForeignKey('parents.id')) parent = relationship("Parent", back_populates="child")
- One-to-many relationshipsIn a one-to-many relationship, a row in one table can be associated with multiple rows in another table. pythonCopy code
class Customer(Base): __tablename__ = 'customers' id = Column(Integer, primary_key=True) orders = relationship("Order", back_populates="customer") class Order(Base): __tablename__ = 'orders' id = Column(Integer, primary_key=True) customer_id = Column(Integer, ForeignKey('customers.id')) customer = relationship("Customer", back_populates="orders")
- Many-to-many relationshipsIn many-to-many relationships, a row in one table can be associated with multiple rows in another table, and vice versa. pythonCopy code
association_table = Table('association', Base.metadata, Column('student_id', Integer, ForeignKey('students.id')), Column('course_id', Integer, ForeignKey('courses.id')) ) class Student(Base): __tablename__ = 'students' id = Column(Integer, primary_key=True) courses = relationship("Course", secondary=association_table, back_populates="students") class Course(Base): __tablename__ = 'courses' id = Column(Integer, primary_key=True) students = relationship("Student", secondary=association_table, back_populates="courses")
Advanced options
- Lazy Loading: Use parameters to specify when to load the associated object. For example, the associated object is loaded immediately on the original query.
lazy
lazy='joined'
- Cascading: Use parameters to specify cascading operations, such as delete.
cascade
- Back Populates: Use parameters to specify a two-way relationship.
back_populates
merit
- Data integrity: By defining relationships and foreign keys, you can ensure the data integrity of your database.
- Ease of use: Using SQLAlchemy’s functions, you can define complex database relationships in Pythonic.
relationship
- Query optimization: After you define relationships, you can more easily execute join queries to optimize data retrieval.
By understanding how to create relationships in SQLAlchemy, you will be able to more effectively simulate complex relationships in the real world, making your database design more robust and flexible. This will also greatly simplify query and data operations, and improve development efficiency.
Please explain in detail how to perform join operations on ChatGPT for relationships and joins
Relationships and joins: How to perform join operations
What is a join operation?
A join operation is used to combine data from two or more tables based on a condition. In SQLAlchemy, you can perform join operations in a variety of ways, including using methods, methods, or specifying join conditions directly in a method.join
outerjoin
filter
The main method
- join: Use the Inner Join method. pythonCopy code
join
query = session.query(User).join(Order).filter(Order.amount > 100)
- outerjoin: Use the Left Outer Join method. pythonCopy code
outerjoin
query = session.query(User).outerjoin(Order).filter(Order.amount == None)
How do I perform a join operation?
- Simple inner join pythonCopy code Here, and are two different model classes that have a foreign key relationship based on them.
from sqlalchemy.orm import joinedload query = session.query(User).join(Order).filter(Order.amount > 100)
User
Order
user_id
- If you need to specify a join condition explicitly, you can do this: pythonCopy code
query = session.query(User).join(Order, User.id == Order.user_id)
- Multi-table joinsYou can also join multiple tables at once. pythonCopy code
query = session.query(User).join(Order).join(Address).filter(Order.amount > 100, Address.city == 'New York')
- Left outer joinIn a left outer join, all records in the left table () are returned even if there are no matching records in the right table (). pythonCopy code
Order
User
query = session.query(User).outerjoin(Order).filter(Order.amount == None)
- Join and select specific columnsIf you are only interested in certain columns in the result set, you can specify those columns explicitly. pythonCopy code
query = session.query(User.name, Order.amount).join(Order).filter(Order.amount > 100)
- Join with
aliasedIf
you need to use the same table multiple times in a query, you can use Create alias. pythonCopy codealiased
from sqlalchemy.orm import aliased OrderAlias = aliased(Order) query = session.query(User, OrderAlias).join(OrderAlias, User.orders)
merit
- Data consolidation: Join operations allow you to combine data from multiple tables for more complex queries and analysis.
- Performance optimization: By using joins wisely, you can improve query performance by reducing the amount of data that needs to be retrieved from the database.
- Flexibility: SQLAlchemy provides a variety of join options, including inner joins, outer joins, and self-joins, giving you the flexibility to meet a variety of query needs.
By learning how to perform join operations in SQLAlchemy, you will be able to retrieve and consolidate data from multiple tables more efficiently. This is a very important aspect of database queries, and mastering it will greatly improve your data manipulation capabilities.
Please explain in detail how transaction management starts and ends transactions in ChatGPT
Transaction management: How to start and end transactions
What is a transaction?
In a database management system, a transaction is a sequence of one or more SQL operations that either succeed or fail to ensure database consistency. In SQLAlchemy, transaction management is usually done through objects.Session
Main components
- Session: In SQLAlchemy’s ORM, objects are the starting point for all database interactions, including transaction management. pythonCopy code
Session
from sqlalchemy.orm import Session session = Session(bind=engine)
How do I start a transaction?
- Automatic Start When you perform a database operation (such as adding, modifying, or deleting records) on an object for the first time, SQLAlchemy automatically starts a new transaction. pythonCopy code In this example, when you call the method, a new transaction starts automatically.
Session
new_user = User(name='John', email='john@example.com') session.add(new_user)
session.add()
- Start manually, or you can use the method to start a transaction manually. pythonCopy code
begin
transaction = session.begin()
How do I end a transaction?
- Commit a transaction uses the method to commit the transaction, which saves all changes and ends the current transaction. pythonCopy code If a transaction commits successfully, all changes made in that transaction are persisted to the database.
commit
session.commit()
- Roll back a transaction If you want to undo all changes made in the current transaction, you can use the method. pythonCopy codeThis undoes all uncommitted changes and ends the transaction.
rollback
session.rollback()
- Manual EndingIf you have started a transaction manually, you can also end it by calling the object’s or method. pythonCopy code or pythonCopy code
transaction
commit
rollback
transaction.commit()
transaction.rollback()
Notes
- Nested transactions: SQLAlchemy supports nested transactions, which means that you can start one transaction inside another. This is usually done through the method.
begin_nested
- Savepoint: Within a transaction, you can set multiple savepoints (Savepoint) so that if the transaction fails, only roll back to a certain state.
- Automatic rollback: In some cases, such as a database disconnection, SQLAlchemy automatically rolls back the current transaction.
- Session lifecycle: In general, there should be a new instance of each request to ensure transaction independence.
Session
merit
- Data consistency: By using transactions, you can ensure that a set of operations either succeed or fail all of them, guaranteeing database consistency.
- Error recovery: With transaction rollback, you can easily recover from errors or failures.
- Concurrency control: Transactions are also used to manage problems that can occur when multiple users access a database concurrently.
By understanding how to start and end transactions in SQLAlchemy, you will be able to manage database operations more effectively and ensure data consistency and integrity. This is a very important part of any application that involves database operations.
Please explain in detail the error handling for transaction management and rollback ChatGPT
Transaction management: error handling and rollback
What is error handling and rollback?
In database transactions, error handling and rollback are two key concepts. When performing a set of operations within a transaction, if any one of them fails, the entire transaction should be rolled back (undone) to guarantee database consistency and integrity.
Error handling
- Catch exceptions When executing a transaction, you should use try-except blocks to catch any exceptions that may occur. pythonCopy code
try: new_user = User(name='John', email='john@example.com') session.add(new_user) session.commit() except Exception as e: print(f"An error occurred: {e}")
- Custom error messages: You can output more specific error messages based on the type of exception you caught. pythonCopy code
from sqlalchemy.exc import IntegrityError, OperationalError try: # ... (transaction code) except IntegrityError: print("Integrity error, possibly due to a constraint violation.") except OperationalError: print("Operational error, possibly due to database connection issues.") except Exception as e: print(f"An unknown error occurred: {e}")
Rollback operation
- Use the
rollback
method After you catch an exception, you should use the RollBack method to undo all uncommitted changes. pythonCopy coderollback
try: # ... (transaction code) session.commit() except Exception as e: session.rollback() print(f"An error occurred: {e}. Transaction rolled back.")
- Using
the with
statement, you can also use the statement and the object’s methods to automatically handle rollbacks. pythonCopy code In this case, if any operation in the transaction fails, the object automatically performs a rollback operation.with
Session
begin
with session.begin(): # ... (transaction code)
Session
- Nested transactions and savepoints In more complex scenarios, you may need to use nested transactions and savepoints. pythonCopy code
try: with session.begin_nested(): # ... (nested transaction code) except Exception as e: session.rollback() print(f"An error occurred in the nested transaction: {e}")
Notes
- Multiple rollbacks: If you call a method multiple times in a transaction, only the first call will be executed.
rollback
- Close Session: After a rollback, you should consider whether you need to close or reset objects, especially in long-running applications.
Session
- Logging: Consider using logging to track the success and failure of transactions, which is useful for debugging and auditing.
merit
- Data security: By properly handling errors and performing rollbacks, you can ensure that the state of your database remains consistent even in the event of an error or failure.
- Serviceability: Use structured error handling and rollback mechanisms to make code easier to maintain and debug.
By understanding how to do error handling and rollback in SQLAlchemy, you will be able to manage database transactions more effectively, especially when errors or exceptions occur. This not only improves the robustness of the application, but also guarantees data consistency and integrity.
Please explain in detail the performance optimization of Eager loading ChatGPT
Performance optimization: Eager loading
What is Eager loading?
In SQLAlchemy, Eager loading is an optimization technique for preloading other objects associated with an object to reduce the number of database queries. This is often used to solve N+1 query problems, where when you get multiple records from one table and access records from other tables associated with it, it can result in a large number of additional database queries.
The main method
- The joinedload method allows you to preload associated objects in a join query. pythonCopy code Here, all orders associated with the user will be loaded in one query.
joinedload
from sqlalchemy.orm import joinedload query = session.query(User).options(joinedload(User.orders)).filter(User.id == 1)
- The SubqueryLoad method allows you to preload associated objects in a separate subquery. pythonCopy code Here, all orders associated with the user will be loaded in a separate subquery.
subqueryload
from sqlalchemy.orm import subqueryload query = session.query(User).options(subqueryload(User.orders)).filter(User.id == 1)
- Using the selectinload method, you can preload associated objects in a query. pythonCopy code Here, all orders associated with the user will be loaded in one query.
selectinload
IN
from sqlalchemy.orm import selectinload query = session.query(User).options(selectinload(User.orders)).filter(User.id == 1)
IN
How do I use Eager Load?
- For a single association, such as a one-to-many relationship, you can simply use , , or . pythonCopy code
joinedload
subqueryload
selectinload
query = session.query(User).options(joinedload(User.orders))
- Multilevel correlation If you have a multi-level association, such as a one-to-many one-to-many relationship, you can use the method. pythonCopy code
joinedload
nested
from sqlalchemy.orm import joinedload query = session.query(User).options(joinedload(User.orders, Order.items))
- Dynamic loadingIf you need to dynamically select a loading strategy based on certain conditions, you can use methods at runtime. pythonCopy code
load
from sqlalchemy.orm import load_only query = session.query(User).options(load_only("name", "email"))
merit
- Performance gains: Eager loading can significantly improve the performance of your application by reducing the number of database queries.
- Simplified code: With Eager loading, you can get all the data you need in one query, simplifying subsequent data processing code.
- Flexibility: SQLAlchemy provides a variety of Eager loading options, allowing you to choose the most appropriate loading strategy for your specific needs.
By understanding and applying Eager loading, you can optimize database queries and improve the performance and responsiveness of your application. This is a very important aspect of database performance tuning.
Please explain in detail the performance optimization of query optimization ChatGPT
Performance optimization: Query optimization
What is query optimization?
Query optimization is a key aspect of database performance tuning to improve the performance of your application by reducing the complexity and number of database queries. In SQLAlchemy, there are several methods and techniques that can be used to optimize queries.
Main methods and techniques
- Selective loading uses methods to load only the fields you actually need, rather than the entire object. pythonCopy code
load_only
from sqlalchemy.orm import load_only query = session.query(User).options(load_only("name", "email"))
- Use indexes to create indexes on key fields of database tables to speed up queries. pythonCopy code
from sqlalchemy import Index index = Index('my_index', User.name)
- Bulk operations use or to bulk insert or update. pythonCopy code
bulk_insert_mappings
bulk_update_mappings
session.bulk_insert_mappings(User, [{'name': 'user1'}, {'name': 'user2'}])
- Query caching uses caching to store frequently accessed query results to reduce database access. pythonCopy code
from sqlalchemy_cache import Cache cache = Cache(session) query = session.query(User).options(cache.cache())
- Optimize subqueries and joins Try to avoid using complex subqueries and joins, or use to analyze query plans and optimize accordingly. sqlCopy code
EXPLAIN
EXPLAIN SELECT * FROM users WHERE name = 'John';
- Paging uses the and methods for query paging to reduce the amount of data returned by a query. pythonCopy code
limit
offset
query = session.query(User).limit(10).offset(20)
- Use EXISTS instead of COUNT when you only need to check if there are records that match the criteria, because the former is usually faster. pythonCopy code
EXISTS
COUNT
from sqlalchemy import exists query = session.query(exists().where(User.name == 'John'))
merit
- Improve performance: By reducing the number and complexity of database queries, you can significantly improve the performance of your application.
- Reduce resource usage: Optimized queries typically use fewer CPU and memory resources.
- Improved responsiveness: Query optimization often results in faster page loads and a smoother user experience for users.
By understanding and applying these query optimization techniques, you can improve the performance of SQLAlchemy and your database to provide faster and more reliable services. This is important for applications of any size.
Please explain in detail the database introspection and the generated auto-generated CREATE statement ChatGPT
Database introspection and generated auto-generated CREATE statements
What is database introspection?
Database introspection is a technique used to obtain detailed information about database schemas, tables, fields, relationships, etc. from a database. In SQLAlchemy, you can use introspection to automatically create or update Python models to match existing database structures.
The main method
- Reflection is the primary method used in SQLAlchemy for database introspection. You can use objects and methods to load information from the database. pythonCopy code In this way, the object will contain information about all tables in the database.
MetaData
reflect
from sqlalchemy import MetaData meta = MetaData() meta.reflect(bind=engine)
meta
- Autoload You can also set it when defining the model to automatically load column information from the database. pythonCopy code
autoload=True
from sqlalchemy import Table my_table = Table('my_table', meta, autoload=True, autoload_with=engine)
Automatically generate CREATE statements
- Use
create_all
Once you have defined the model, you can use the CREATE method to automatically generate a CREATE statement and create the database tables. pythonCopy code This generates the appropriate CREATE statements for all models defined in the class and executes them to create the table.create_all
Base.metadata.create_all(engine)
Base
- Generate SQL Statements If you want to view the generated SQL CREATE statements without executing them, you can use the method. pythonCopy code: This will output the CREATE TABLE statement corresponding to the model.
string
from sqlalchemy.schema import CreateTable print(CreateTable(User.__table__).compile(engine))
User
merit
- Synchronize models and databases: By using database introspection, you can ensure that Python models and database schemas are always in sync.
- Less manual effort: Automatically generating CREATE statements reduces the effort to manually create and maintain a database schema.
- Fewer errors: Auto-generated SQL statements are often more accurate than manually written, reducing the likelihood of errors.
By understanding database introspection and how to automatically generate CREATE statements, you can manage and maintain the database schema more effectively, while also reducing manual effort and errors. This is very useful in database design and maintenance.
Please explain in detail the best practices and advanced topics of security in ChatGPT
Best practices and advanced topics: Security
What is database security?
Database security involves measures to protect a database and its contents from unauthorized access and manipulation. When using SQLAlchemy or any other database access library, it is important to consider security.
Primary security measures
- SQL injection protectionSQLAlchemy’s query constructor is designed to automatically escape all SQL parameters, reducing the risk of SQL injection. Try to avoid using raw SQL queries. pythonCopy code
# 推荐 session.query(User).filter_by(name='John') # 不推荐 session.execute("SELECT * FROM users WHERE name = 'John';")
- The principle of least privilege assigns as few permissions as possible to database users. For example, if a service only needs to read data, it should not be given write permissions.
- Use environment variables to store database credentials, and do not hard-code database credentials in your code. Use environment variables or key management services to store this information. pythonCopy code
import os db_url = os.environ.get('DATABASE_URL')
- Data encryption uses SSL/TLS to encrypt communication with the database. Also, consider using field-level encryption to protect sensitive data.
- Auditing and logging enables audit logs for databases and applications to track down security events as they occur.
- Input validation always validates and cleans up user input before it is used for database queries. pythonCopy code
from validate_email_address import validate_email if validate_email(user_email): session.query(User).filter_by(email=user_email)
Advanced themes
- Row-Level Security implements row-level security at the database level to restrict which users can access which rows of data.
- Use the security mode of the ORMSQLAlchemy provides a “safe mode” that disables certain potentially unsafe operations, such as dynamic field names.
- Database firewall: Use a database firewall to restrict which IP addresses can access your database.
- Regular Security Audits Conduct regular security audits to detect and fix any potential security vulnerabilities.
merit
- Data protection: By implementing these security best practices, you can protect your data more effectively.
- Compliance: Many industries and regions have strict data protection and privacy regulations, and following these best practices can help with compliance.
- Reduce risk: By preventing unauthorized access and manipulation, you can significantly reduce the risk of data breaches and other security incidents.
By understanding and applying these best practices and advanced topics for database security, you can not only protect your data, but also improve the overall security of your application. This is a very important part of any database application development and maintenance.